Topic 2 Probability and DAGs
Learning Goals
- Appreciate the importance of starting analysis from prior/expert knowledge
- Simulate data corresponding to a causal DAG
- Use simulations to verify the marginal/conditional independence and dependence relationships implied by DAG structures
- Develop notions of causal and non-causal associations in the context of graphs
Goal 1
In your groups, discuss your responses to the pre-class question about the figure below (which came from this article from Nature Pediatric Research).
- What arrows do all of you agree on?
- Do you disagree on any arrows?
- If the question of interest is how screen time affects the risk of childhood obesity, what variables and arrows do you think are missing?
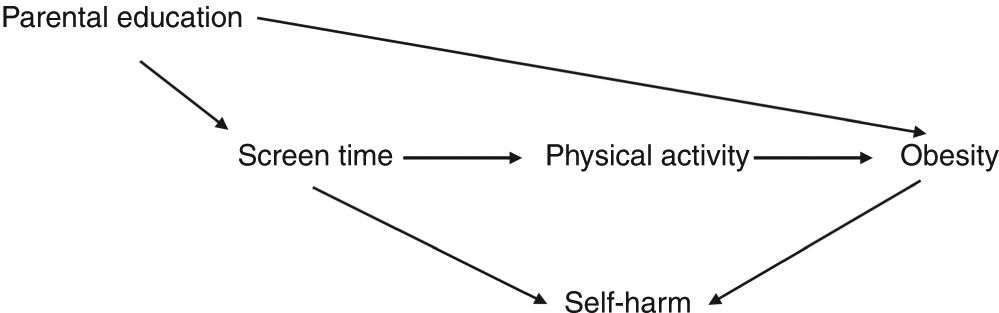
If you have time left, pick your favorite example of conditional independence and conditional dependence from your answers to the pre-class questions.
Discussion
Download this Rmd file to start from.
library(ggplot2)
library(dplyr)
An introduction to the rbinom
function:
# 4 different people each flip a fair coin once
rbinom(4, size = 1, prob = 0.5)
# 4 different people flip loaded coins
# First 2 flip a coin with P(Heads) = 0.9
# Second 2 flip a coin with P(Heads) = 0.2
rbinom(4, size = 1, prob = c(0.9, 0.9, 0.2, 0.2))
# Before you run this code, what will the result be?
rbinom(2, size = 1, prob = c(1, 0))
How can we simulate X -> Y for binary X and Y?
# set.seed() ensures reproducible random numbers
set.seed(2020)
# Set sample size
1e5
n <-# Generate a binary variable X for all n cases
rbinom(n, size = 1, prob = 0.5)
X <- rbinom(n, size = 1, prob = 0.5) Y <-
Are the X and Y we just simulated independent or dependent? Let’s make a plot to see:
# We put X and Y in a dataset using data.frame()
# They are turned into categorical variables using factor()
data.frame(X = factor(X), Y = factor(Y))
sim_data <-
# Proportional bar plot
ggplot(sim_data, aes(x = X, fill = Y)) +
geom_bar(position = "fill")
How can we make Y dependent on X?
# Generate probabilities that Y=1 that depend on the value of X
dplyr::case_when(
p_Y <-==1 ~ 0.8,
X==0 ~ 0.3
X
)
# Simulate Y using p_Y
rbinom(n, size = 1, prob = p_Y)
Y <-
# Put X, Y, and p_Y into a dataset
data.frame(X = factor(X), Y = factor(Y), p_Y = p_Y)
sim_data <-
# Check that p_Y = 0.8 when X is 1
%>%
sim_data filter(X=="1")
# Check that p_Y = 0.3 when X is 0
%>%
sim_data filter(X=="0")
# Proportional bar plot
ggplot(sim_data, aes(x = X, fill = Y)) +
geom_bar(position = "fill")
How can we make a variable Z dependent on 2 causes: X and Y?
rbinom(n, size = 1, prob = 0.5)
X <- rbinom(n, size = 1, prob = 0.5)
Y <- dplyr::case_when(
p_Z <-==1 & Y==1 ~ 0.8,
X==0 & Y==1 ~ 0.3,
X==1 & Y==0 ~ 0.5,
X==0 & Y==0 ~ 0.9
X
) rbinom(n, size = 1, prob = p_Z)
Z <-
# Put X, Y, and Z into a dataset
data.frame(X = factor(X), Y = factor(Y), Z = factor(Z))
sim_data <-
# If we wanted to save datasets filtered on Z (Why?)
sim_data %>% filter(Z=="0")
sim_data_filt_z0 <- sim_data %>% filter(Z=="1") sim_data_filt_z1 <-
R Exercises
Exercise 1
Simulate data for a chain X -> Y -> Z where all variables are binary. Make plots to show the following properties:
- X and Z are marginally dependent
- X and Z are conditionally independent given Y
Describe how your simulation would change if you wanted to check the more general property of chains that two variables are conditionally independent given any variables in between them. (You don’t actually need to implement this simulation but can if you have time.)
Exercise 2
Simulate data for a fork Y <- X -> Z where all variables are binary. Make plots to show the following properties:
- Y and Z are marginally dependent
- Y and Z are conditionally independent given X
Exercise 3
Simulate data for a collider X -> Z <- Y where all variables are binary. Make plots to show the following properties:
- X and Y are marginally independent
- X and Y are conditionally dependent given Z
Describe how your simulation would change if you wanted to check the more general property of colliders that X and Y become dependent conditional on Z and/or any descendants of Z. (You don’t actually need to implement this simulation but can if you have time.)
Conceptual Exercises
Exercise 4
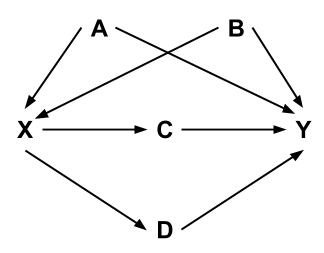
In the causal diagram above, let X be a treament and Y be an outcome of interest.
- What would you say are the “causal paths” between X and Y? What would you say are the “non-causal paths”?
- Thinking about the marginal and conditional independence and dependence relations we’ve discussed, how might we restrict the influence of these non-causal paths?